- This private slot is used to move the shot while it is in the air, using a QTimer. Private: void paintShot( QPainter. ); This private function paints the shot. QRect shotRect const; This private function returns the shot's enclosing rectangle if one is in the air; otherwise the returned rectangle is undefined.
- One key and distinctive feature of Qt framework is the use of signals and slots to connect widgets and related actions. But as powerful the feature is, it may look compelling to a lot of developers not used to such a model, and it may take some time at the beginning to get used to understand how to use signals and slots properly.
We declare several private slots: The setShape slot changes the RenderArea's shape, the setPen and setBrush slots modify the widget's pen and brush, and the setAntialiased and setTransformed slots modify the widget's respective properties. RenderArea Class Implementation. In the constructor we initialize some of the widget's variables. In this tutorial, we will learn about StatusBar. The QStatusBar class provides a horizontal bar suitable for presenting status information. In this example, we'll start from MainWindow class. We need to add menu actions. In this example, we'll add just one menu (MyMenuAction) to trigger action.
Home All Classes Main Classes Annotated Grouped Classes Functions |
In this example we introduce a timer to implement animated shooting.
Slots and signals must have same parameters. Otherwise, the connection will not occur. Not only for connection, slot function must have same parameters with signal. For example, this sample doesn’t work: QObject::connect(ui.comboBox, SIGNAL (activated(int)), this, SLOT (onComboboxActivated)); But it works.
- t11/lcdrange.h contains the LCDRangeclass definition.
- t11/lcdrange.cpp contains the LCDRangeimplementation.
- t11/cannon.h contains the CannonField classdefinition.
- t11/cannon.cpp contains the CannonFieldimplementation.
- t11/main.cpp contains MyWidget and main.
Line-by-line Walkthrough
t11/cannon.h
The CannonField now has shooting capabilities.
Calling this slot will make the cannon shoot if a shot is not in the air.
This private slot is used to move the shot while it is in the air,using a QTimer.
This private function paints the shot.
This private function returns the shot's enclosing rectangle ifone is in the air; otherwise the returned rectangle is undefined.
These private variables contain information that describes the shot. ThetimerCount keeps track of the time passed since the shot was fired.The shoot_ang is the cannon angle and shoot_f is the cannon forcewhen the shot was fired.
t11/cannon.cpp
We include the math library because we need the sin() and cos() functions.
We initialize our new private variables and connect the QTimer::timeout() signal to our moveShot() slot. We'll move theshot every time the timer times out.
This function shoots a shot unless a shot is in the air. The timerCountis reset to zero. The shoot_ang and shoot_f are set to the currentcannon angle and force. Finally, we start the timer.
moveShot() is the slot that moves the shot, called every 50milliseconds when the QTimer fires.
Its tasks are to compute the new position, repaint the screen with theshot in the new position, and if necessary, stop the timer.
First we make a QRegion that holds the old shotRect(). A QRegionis capable of holding any sort of region, and we'll use it here tosimplify the painting. ShotRect() returns the rectangle where theshot is now - it is explained in detail later.
Then we increment the timerCount, which has the effect of moving theshot one step along its trajectory.
Next we fetch the new shot rectangle.
If the shot has moved beyond the right or bottom edge of the widget, westop the timer or we add the new shotRect() to the QRegion.
Finally, we repaint the QRegion. This will send a single paint eventfor just the one or two rectangles that need updating.
The paint event function has been split in two since the previouschapter. Now we fetch the bounding rectangle of the region thatneeds painting, check whether it intersects either the cannon and/orthe shot, and if necessary, call paintCannon() and/or paintShot().
This private function paints the shot by drawing a black filled rectangle.
We leave out the implementation of paintCannon(); it is the same asthe paintEvent() from the previous chapter.
This private function calculates the center point of the shot and returnsthe enclosing rectangle of the shot. It uses the initial cannon force andangle in addition to timerCount, which increases as time passes.
The formula used is the classical Newtonian formula for frictionlessmovement in a gravity field. For simplicity, we've chosen todisregard any Einsteinian effects.
We calculate the center point in a coordinate system where ycoordinates increase upward. After we have calculated the centerpoint, we construct a QRect with size 6x6 and move its center point tothe point calculated above. In the same operation we convert thepoint into the widget's coordinate system (see TheCoordinate System).
The qRound() function is an inline function defined in qglobal.h (includedby all other Qt header files). qRound() rounds a double to the closestinteger.
t11/main.cpp
The only addition is the Shoot button.
In the constructor we create and set up the Shoot button exactly like wedid with the Quit button. Note that the first argument to the constructoris the button text, and the third is the widget's name.
Connects the clicked() signal of the Shoot button to the shoot() slotof the CannonField.
Behavior
The cannon can shoot, but there's nothing to shoot at.
(See Compiling for how to create amakefile and build the application.)
Exercises
Make the shot a filled circle. Hint: QPainter::drawEllipse() mayhelp.
Change the color of the cannon when a shot is in the air.
You're now ready for Chapter 12.
[Previous tutorial][Next tutorial][Main tutorial page]
A window flag is either a type or a hint. A type is used to specify various window-system properties for the widget. A widget can only have one type, and the default is Qt::Widget. However, a widget can have zero or more hints. The hints are used to customize the appearance of top-level windows.
A widget's flags are stored in a Qt::WindowFlags type which stores an OR combination of the flags.
Screenshot of the Window Flags example
The example consists of two classes:
ControllerWindow
is the main application widget that allows the user to choose among the available window flags, and displays the effect on a separate preview window.PreviewWindow
is a custom widget displaying the name of its currently set window flags in a read-only text editor.
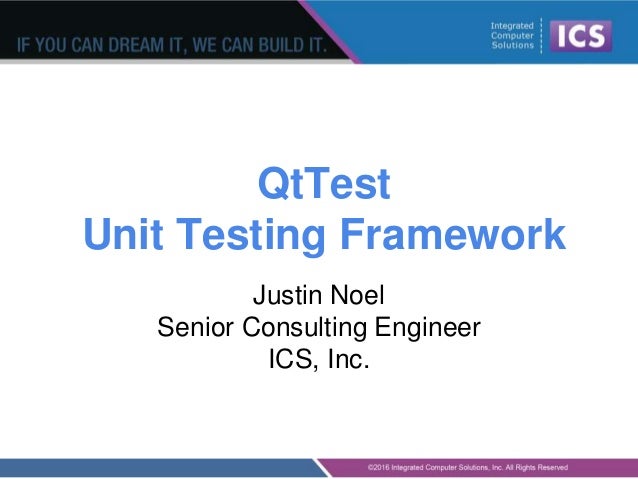
We will start by reviewing the ControllerWindow
class, then we will take a look at the PreviewWindow
class.
ControllerWindow Class Definition
The ControllerWindow
class inherits QWidget. The widget allows the user to choose among the available window flags, and displays the effect on a separate preview window.
We declare a private updatePreview()
slot to refresh the preview window whenever the user changes the window flags.
We also declare several private functions to simplify the constructor: We call the createTypeGroupBox()
function to create a radio button for each available window type, using the private createButton()
function, and gather them within a group box. In a similar way we use the createHintsGroupBox()
function to create a check box for each available hint, using the private createCheckBox()
function.
In addition to the various radio buttons and checkboxes, we need an associated PreviewWindow
to show the effect of the currently chosen window flags.

ControllerWindow Class Implementation
In the constructor we first create the preview window. Then we create the group boxes containing the available window flags using the private createTypeGroupBox()
and createHintsGroupBox()
functions. In addition we create a Quit button. We put the button and a stretchable space in a separate layout to make the button appear in the WindowFlag
widget's right bottom corner.
Qt Private Slot Example For Real
Finally, we add the button's layout and the two goup boxes to a QVBoxLayout, set the window title and refresh the preview window using the updatePreview()
slot.
The updatePreview()
slot is called whenever the user changes any of the window flags. First we create an empty Qt::WindowFlagsflags
, then we determine which one of the types that is checked and add it to flags
.
Qt Private Slot Example Software
We also determine which of the hints that are checked, and add them to flags
using an OR operator. We use flags
to set the window flags for the preview window.
We adjust the position of the preview window. The reason we do that, is that playing around with the window's frame may on some platforms cause the window's position to be changed behind our back. If a window is located in the upper left corner of the screen, parts of the window may not be visible. So we adjust the widget's position to make sure that, if this happens, the window is moved within the screen's boundaries. Finally, we call QWidget::show() to make sure the preview window is visible.
The private createTypeGroupBox()
function is called from the constructor.
First we create a group box, and then we create a radio button (using the private createRadioButton()
function) for each of the available types among the window flags. We make Qt::Window the initially applied type. We put the radio buttons into a QGridLayout and install the layout on the group box.
We do not include the default Qt::Widget type. The reason is that it behaves somewhat different than the other types. If the type is not specified for a widget, and it has no parent, the widget is a window. However, if it has a parent, it is a standard child widget. The other types are all top-level windows, and since the hints only affect top-level windows, we abandon the Qt::Widget type.
The private createHintsGroupBox()
function is also called from the constructor.
Qt Private Slot Examples
Again, the first thing we do is to create a group box. Then we create a checkbox, using the private createCheckBox()
function, for each of the available hints among the window flags. We put the checkboxes into a QGridLayout and install the layout on the group box.
The private createCheckBox()
function is called from createHintsGroupBox()
.
We simply create a QCheckBox with the provided text, connect it to the private updatePreview()
slot, and return a pointer to the checkbox.
In the private createRadioButton()
function it is a QRadioButton we create with the provided text, and connect to the private updatePreview()
slot. The function is called from createTypeGroupBox()
, and returns a pointer to the button.
PreviewWindow Class Definition
The PreviewWindow
class inherits QWidget. It is a custom widget that displays the names of its currently set window flags in a read-only text editor. It is also provided with a QPushbutton that closes the window.
We reimplement the constructor to create the Close button and the text editor, and the QWidget::setWindowFlags() function to display the names of the window flags.
Qt Private Slot Example Value
PreviewWindow Class Implementation
In the constructor, we first create a QTextEdit and make sure that it is read-only.
We also prohibit any line wrapping in the text editor using the QTextEdit::setLineWrapMode() function. The result is that a horizontal scrollbar appears when a window flag's name exceeds the width of the editor. This is a reasonable solution since we construct the displayed text with built-in line breaks. If no line breaks were guaranteed, using another QTextEdit::LineWrapMode would perhaps make more sense.
Then we create the Close button, and put both the widgets into a QVBoxLayout before we set the window title.
In our reimplementation of the setWindowFlags()
function, we first set the widgets flags using the QWidget::setWindowFlags() function. Then we run through the available window flags, creating a text that contains the names of the flags that matches the flags
parameter. Finally, we display the text in the widgets text editor.
Files:
© 2019 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.